+
+// E X P O R T
+
+module.exports = exports = () => html`
+
+
+
+
+
The requested page could not be found. Here is the image located at lbry://404 to console you.
+
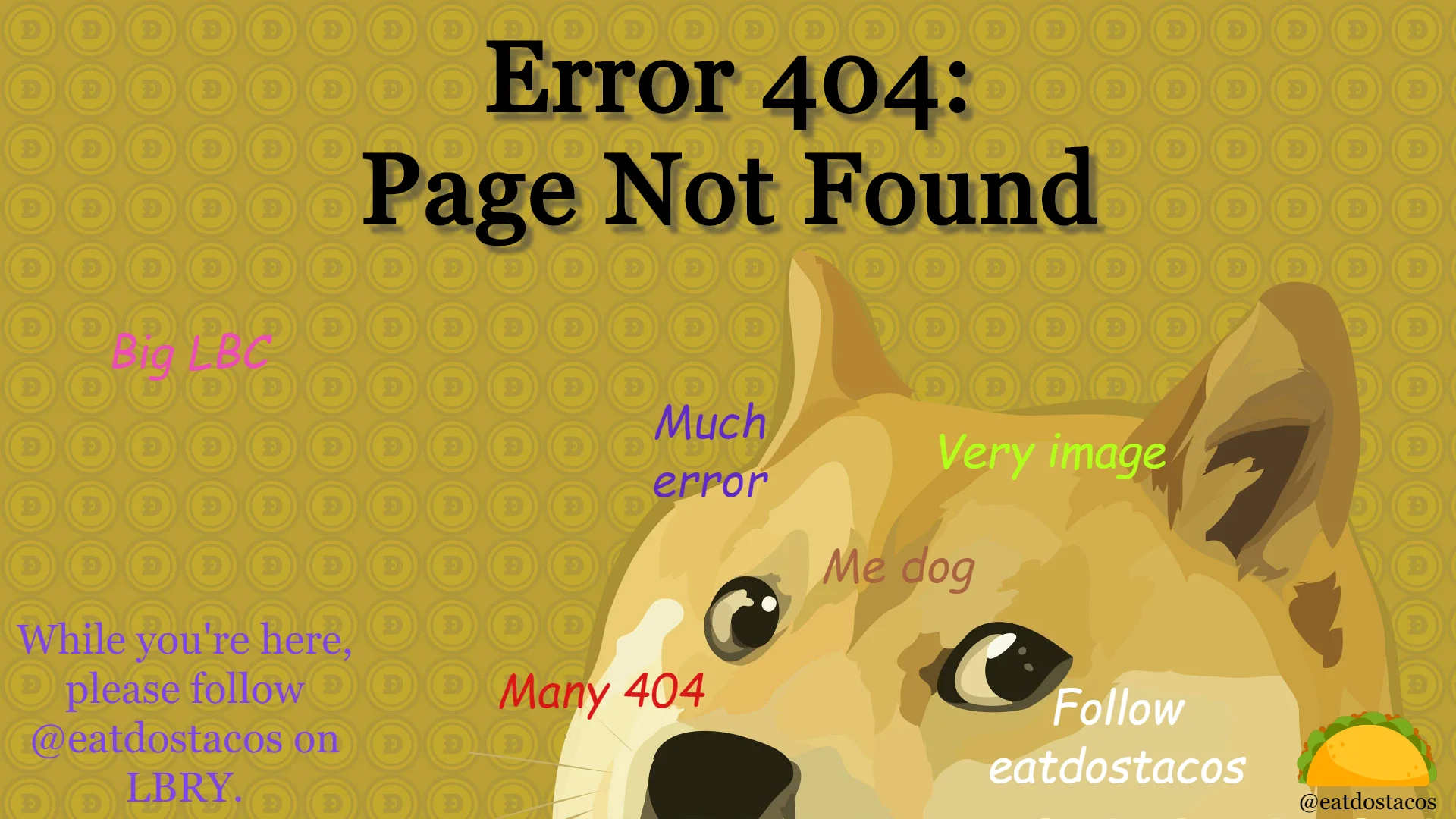
+
Think something should be here? Let us know by raising an issue.
+
+
+
+`;
diff --git a/app/views/api.js b/app/views/api.js
index 4aa87e1..41b3171 100644
--- a/app/views/api.js
+++ b/app/views/api.js
@@ -1,12 +1,23 @@
"use strict";
+
+
+// P A C K A G E S
+
import asyncHtml from "choo-async/html";
import dedent from "dedent";
-import redirectOr404 from "../modules/redirectOr404";
-import headerBlockchain from "../components/api/header-blockchain";
-import headerSdk from "../components/api/header-sdk";
+import { require as local } from "app-root-path";
+
+// V A R I A B L E S
const fetch = require("make-fetch-happen").defaults({ cacheManager: "./cache" });
+const headerBlockchain = local("app/components/api/header-blockchain").default;
+const headerSdk = local("app/components/api/header-sdk").default;
+const redirects = local("app/data/redirects.json");
+
+
+
+// E X P O R T
module.exports = exports = state => parseApiFile(state.params.wildcard).then(response => {
/*
@@ -41,23 +52,37 @@ module.exports = exports = state => parseApiFile(state.params.wildcard).then(res
`;
}).catch(() => {
- redirectOr404(state.href);
+ const redirectUrl = redirects[state.href];
+
+ return asyncHtml`
+
+
+
+
+
+
Redirecting you to ${redirectUrl}
+
+
+
+
+
+ `;
});
-
// H E L P E R S
-function createApiHeader(slug) {
- switch (slug) {
- case "sdk":
- return headerSdk();
- case "blockchain":
- return headerBlockchain();
- }
-}
-
function createApiContent(apiDetails) {
const apiContent = [];
@@ -85,6 +110,19 @@ function createApiContent(apiDetails) {
return apiContent;
}
+function createApiHeader(slug) {
+ switch(slug) {
+ case "blockchain":
+ return headerBlockchain();
+
+ case "sdk":
+ return headerSdk();
+
+ default:
+ break;
+ }
+}
+
function createApiSidebar(apiDetails) {
const apiSidebar = [];
@@ -104,18 +142,26 @@ function createApiSidebar(apiDetails) {
function parseApiFile(urlSlug) {
let apiFileLink;
- //checks below are related to rate limits, both URLs should return the same content
- if (urlSlug === "sdk") apiFileLink = process.env.NODE_ENV === "development" ?
- "https://rawgit.com/lbryio/lbry/master/docs/api.json" :
- "https://cdn.rawgit.com/lbryio/lbry/master/docs/api.json"
- ;
+ // checks below are related to rate limits, both URLs should return the same content
- if (urlSlug === "blockchain") apiFileLink = process.env.NODE_ENV === "development" ?
- "https://rawgit.com/lbryio/lbrycrd/add_api_docs_scripts/contrib/devtools/generated/api_v1.json" :
- "https://cdn.rawgit.com/lbryio/lbrycrd/add_api_docs_scripts/contrib/devtools/generated/api_v1.json"
- ;
+ switch(true) {
+ case (urlSlug === "blockchain"):
+ apiFileLink = process.env.NODE_ENV === "development" ?
+ "https://rawgit.com/lbryio/lbrycrd/add_api_docs_scripts/contrib/devtools/generated/api_v1.json" :
+ "https://cdn.rawgit.com/lbryio/lbrycrd/add_api_docs_scripts/contrib/devtools/generated/api_v1.json";
+ break;
- if (!apiFileLink) return Promise.reject(new Error("Failed to fetch API docs")); // TODO: Error handling
+ case (urlSlug === "sdk"):
+ apiFileLink = process.env.NODE_ENV === "development" ?
+ "https://rawgit.com/lbryio/lbry/master/docs/api.json" :
+ "https://cdn.rawgit.com/lbryio/lbry/master/docs/api.json";
+ break;
+
+ default:
+ break;
+ }
+
+ if (!apiFileLink) return Promise.reject(new Error("Failed to fetch API docs"));
return fetch(apiFileLink).then(() => fetch(apiFileLink, {
cache: "no-cache" // forces a conditional request
diff --git a/app/views/home.js b/app/views/home.js
index 7f706bb..14eff9e 100644
--- a/app/views/home.js
+++ b/app/views/home.js
@@ -1,7 +1,19 @@
"use strict";
+
+
+// P A C K A G E S
+
import html from "choo/html";
-import linkGrid from "../components/link-grid";
+import { require as local } from "app-root-path";
+
+// V A R I A B L E
+
+const linkGrid = local("app/components/link-grid").default;
+
+
+
+// E X P O R T
module.exports = exports = () => html`
diff --git a/app/views/redirect.js b/app/views/redirect.js
index bbd22f2..a8584e3 100644
--- a/app/views/redirect.js
+++ b/app/views/redirect.js
@@ -11,12 +11,12 @@ import fs from "graceful-fs";
import html from "choo/html";
import path from "path";
import { require as local } from "app-root-path";
-import redirectOr404 from "../modules/redirectOr404";
import raw from "choo/html/raw";
// V A R I A B L E S
const numberRegex = /^[0-9]/g;
+const redirect404 = local("app/modules/redirect-404");
const md = require("markdown-it")({
html: true,
@@ -38,6 +38,8 @@ const md = require("markdown-it")({
}
});
+
+
// E X P O R T
module.exports = exports = (state, emit) => { // eslint-disable-line
@@ -45,9 +47,8 @@ module.exports = exports = (state, emit) => { // eslint-disable-line
if (state.route === "resources/*") path = `resources/${state.params.wildcard}`;
else path = state.params.wildcard;
- if (!fs.existsSync(`./documents/${path}.md`)) {
- return redirectOr404(state.href);
- }
+ if (!fs.existsSync(`./documents/${path}.md`))
+ return redirect404(state);
const markdownFile = fs.readFileSync(`./documents/${path}.md`, "utf-8");
const markdownFileDetails = fm(markdownFile);
@@ -106,9 +107,10 @@ function partialFinder(markdownBody) {
const filename = decamelize(partial, "-").replace("<", "").replace("/>", "").trim();
const fileExistsTest = exists(`./app/components/${filename}.js`); // `local` results in error if used here and file !exist
- if (!fileExistsTest) {
+ if (!fileExistsTest)
markdownBody = markdownBody.replace(partial, "");
- } else {
+
+ else {
const partialFunction = require(path.join(__dirname, "..", `./components/${filename}.js`));
if (filename === "glossary-toc") markdownBody = markdownBody.replace(partial, partialFunction);
@@ -119,15 +121,13 @@ function partialFinder(markdownBody) {
return markdownBody;
}
-
function wikiFinder(markdownBody) {
return markdownBody.replace(/\[\[([\w\s/-]+)\]\]/g, (match, p1) => {
- const label = p1.trim(),
- href = encodeURI("/glossary#" + label.replace(/\s+/g, "-"));
+ const label = p1.trim();
+ const url = encodeURI("/glossary#" + label.replace(/\s+/g, "-"));
return label ?
- `
${label}` :
+ `
${label}` :
match.input;
- }
- );
+ });
}